Hey fellas, hope you are doing great! So, in my last article, I had explained to you all the very basics of encryption and decryption and a few types of encryption.
So in today’s article I will talk about AES and how to implement it.
Let’s get going guyzz…
- Technology: python 3.8
Python package : pycryptodome 3.9.7
To install python, check this link: https://www.python.org/downloads/windows/
Once you are through with the installation, please check whether it is successfully installed by the command prompt key.
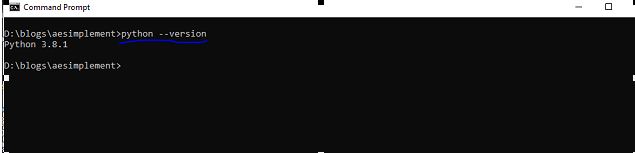
To install pycryptodome please follow bellow steps :
Step 1. Create a project with virtualenv you can change AES implementation with your project name to know more about virtual you can follow this link: https://docs.python.org/3/tutorial/venv.html
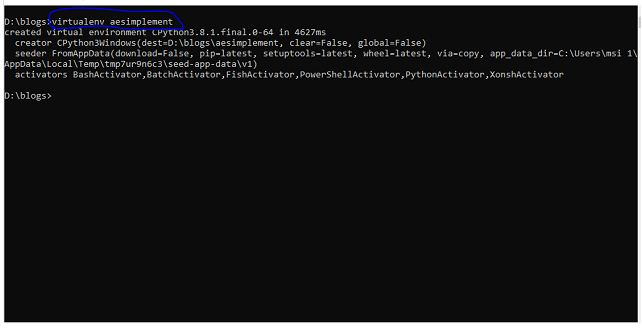
Step 2. Move to the project folder and activate you virtual env
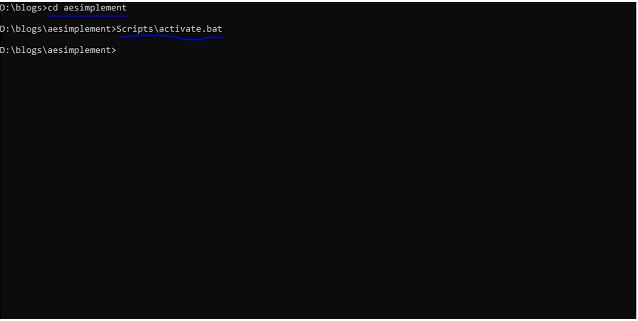
Step 3: Install pycryptodome using pip or whatever pip version you have available.
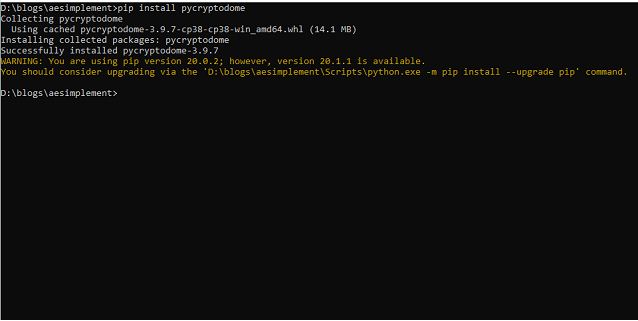
Steps for AES implementation.
Modes of AES :
A. ECB (Electronic Code Book) Mode of Operation
B. CBC (Cipher Block Chaining) Mode of Operation
C. CFB (Cipher FeedBack) Mode of Operation
D. OFB (Output FeedBack) Mode of Operation
E. CTR (Counter) Mode of Operation
If you wanna learn more about modes of AES, refer to this link: https://www.researchgate.net/publication/236656798_MODES_OF_OPERATION_OF_THE_AES_ALGORITHM#:~:text=Back%20in%202001%2C%20five%20modes,)%20and%20CTR%20(Counter).
**We will use CBC Mode of Operation in this program
now in python code, we will create a function to encrypt data.
**import basic required packages.
To encrypt data we need secret key and iv(initialization vector) link to know more about iv https://en.wikipedia.org/wiki/Initialization_vector
Key should have length of 32 characters so it will be the hash function to generate 32 bit of any secret key.
eg key is “this is a md5 secret key“
to create md of key
key = 'this is a md5 key'
md5_key_text = hashlib.md5(key.encode())
md5_hex_key = md5_key_text.hexdigest()
key = bytearray(md5_hex_key,'US-ASCII')
md5 of key “this is a md5 secret key“
fe089bcb917802b0ef9decd7565de3ec
python code to generate iv of 128 bit
iv = ''.join([chr(random.randint(0, 127)) for i in range(16)])
iv = bytearray(iv,'US-ASCII')
HOW TO ENCRYPT DATA?
I have created this function in such a way that it will help us to encrypt file clearly. In this case key,iv and data must be converted in byte and this function will return a bytearray.
def encrypt_data(key,iv,data):
aes = AES.new(key, AES.MODE_CBC, iv)
encd = ''
encd = bytearray()
data = bytearray(data,'US-ASCII')
i = 0
while True:
st = i16 se = ((i+1)16)
ecd = data[st:se]
n = len(ecd)
i += 1
if n == 0:
break
elif n % 16 != 0:
padded=' ' * (16 - n % 16)
byte_padded=(bytearray(padded,'US-ASCII'))
ecd += byte_padded
encd += aes.encrypt(ecd)
return encd
HOW TO DECRYPT DATA?
def decrypt_data(key,iv,data):
des= AES.new(key, AES.MODE_CBC, iv)
decd = bytearray()
i = 0
while True:
st = i2048 se =
dcd = data[st:se]
n = len(dcd)
i += 1
if n == 0:
break
decd += des.decrypt(dcd)
return decd.decode("US-ASCII")
LET’S LEARN ABOUT THE FULL PROGRAM!
import hashlib
import random
from Crypto.Cipher import AES
key = 'this is a md5 key'
md5_key_text = hashlib.md5(key.encode())
md5_hex_key = md5_key_text.hexdigest()
key = bytearray(md5_hex_key,'US-ASCII')
def encrypt_data(key,iv,data):
aes = AES.new(key, AES.MODE_CBC, iv)
encd = ''
encd = bytearray()
data = bytearray(data,'US-ASCII')
i = 0
while True:
st = i16 se = ((i+1)16)
ecd = data[st:se]
n = len(ecd)
i += 1
if n == 0:
break
elif n % 16 != 0:
padded=' ' * (16 - n % 16)
byte_padded=(bytearray(padded,'US-ASCII'))
ecd += byte_padded
encd += aes.encrypt(ecd)
return encd
def decrypt_data(key,iv,data):
des= AES.new(key, AES.MODE_CBC, iv)
decd = bytearray()
i = 0
while True:
st = i2048 se = ((i+1)*2048)
dcd = data[st:se]
n = len(dcd)
i += 1
if n == 0:
break
decd += des.decrypt(dcd)
return decd.decode("US-ASCII")
iv = '1234567890123456'
iv = bytearray(iv,'US-ASCII')
data_to_encrypt = 'my name is anthony and i am on a nuclear project this is most secret mission'
res = encrypt_data(key,iv,data_to_encrypt)
print('encrypted data is ')
print(res)
print('\n\n')
dres = decrypt_data(key,iv,res)
print(dres)
I hope you had a clear idea about the functionality of encryption and decryption. And now, you can easily go to encrypting your data and keep it safe!